Flutter Row – Expanded Children
You can make the children of Row widget, expand to the available horizontal space. All you need to do is, wrap each child of Row widget around Expanded widget.
Example: Expanded Children in Row Widget
In the following example, we have taken three children for Row widget and each of them is wrapped with Expanded widget.
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(MaterialApp(
home: MyApp(),
));
}
class MyApp extends StatefulWidget {
@override
_State createState() => _State();
}
class _State extends State<MyApp> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Tutorial - googleflutter.com'),
),
body: Row(
children: <Widget>[
Expanded(
child: Icon(
Icons.access_alarm,
size: 80,
),
),
Expanded(
child: Icon(
Icons.account_circle,
size: 80,
),
),
Expanded(
child: Icon(
Icons.save,
size: 80,
),
),
],
),
);
}
}
Screenshot
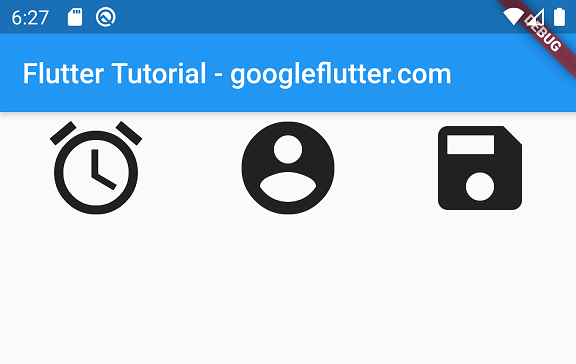
Example 2: Some Expanded Children in Row Widget
Let us take four children and keep only two of them wrapped with Expanded widget.
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(MaterialApp(
home: MyApp(),
));
}
class MyApp extends StatefulWidget {
@override
_State createState() => _State();
}
class _State extends State<MyApp> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Tutorial - googleflutter.com'),
),
body: Row(
children: <Widget>[
Icon(
Icons.access_alarm,
size: 60,
),
Icon(
Icons.account_circle,
size: 60,
),
Expanded(
child: Icon(
Icons.save,
size: 60,
),
),
Expanded(
child: Icon(
Icons.volume_up,
size: 60,
),
),
],
),
);
}
}
Screenshot
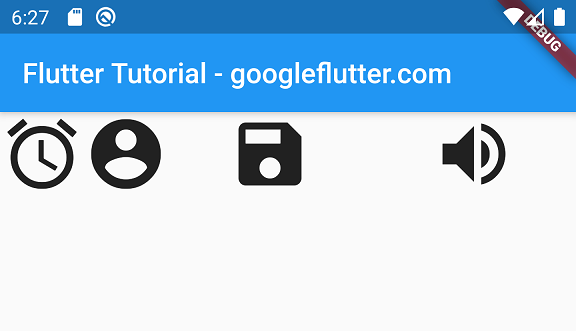
Summary
In this Flutter Tutorial, we learned how to make the children of Row widget occupy equal space in a row, using Expanded widget, with the help of well detailed example Flutter Application.