Get TextField Value in Flutter
In this tutorial, you will learn how to get the value entered by user in TextField widget.
You have to use TextEditingController for controller property of TextField.
Sample Code Snippet
Following is a simple code snippet demonstrating on how to attach a controller to TextField.
//inside state class
TextEditingController nameController = TextEditingController();
TextField(
controller: nameController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'User Name',
),
),
After this, you can use text property of the controller to get the value entered in the TextField, as shown below.
nameController.text
Example
In this example Flutter Application, we take two TextFields and a RaisedButton. After entering user name and password, the user clicks on the button. In the onPressed () property of button, we shall get the values entered in the text fields and print them to console.
To recreate this example, create a Flutter Application and replace main.dart with the following main.dart code.
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(MaterialApp(
home: MyApp(),
));
}
class MyApp extends StatefulWidget {
@override
_State createState() => _State();
}
class _State extends State<MyApp> {
TextEditingController nameController = TextEditingController();
TextEditingController passwordController = TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Tutorial - googleflutter.com'),
),
body: Padding(
padding: EdgeInsets.all(10),
child: Column(
children: <Widget>[
Padding(
padding: EdgeInsets.all(10),
child: TextField(
controller: nameController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'User Name',
),
),
),
Padding(
padding: EdgeInsets.all(10),
child: TextField(
obscureText: true,
controller: passwordController,
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'Password',
),
),
),
RaisedButton(
textColor: Colors.white,
color: Colors.blue,
child: Text('Login'),
onPressed: (){
print(nameController.text);
print(passwordController.text);
},
)
],
)
)
);
}
}
Run this application, and you should get UI similar to that of shown in the following screenshot.
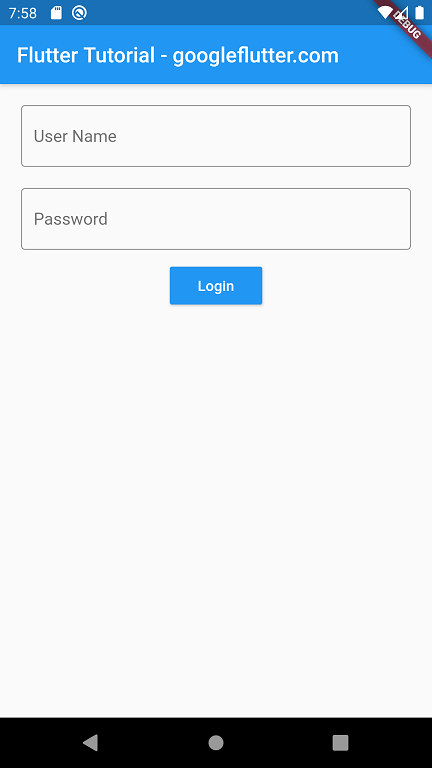
Enter some text in the text fields and click on the Login button.
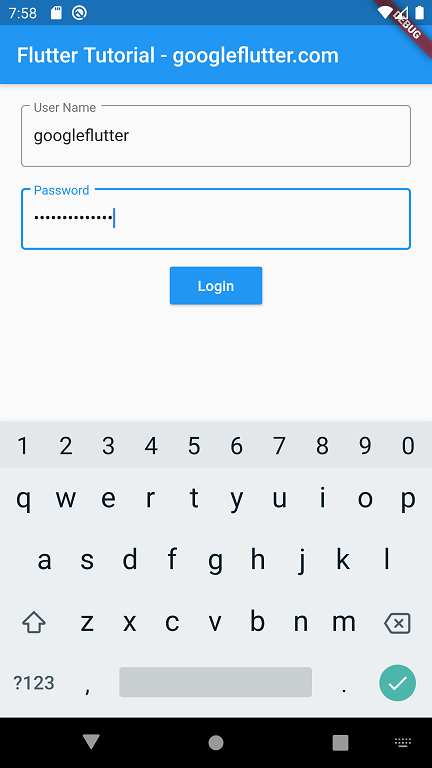
You should see the values, you entered in the text fields, printed to the console as shown below.
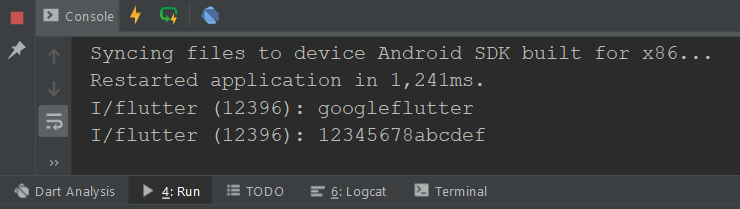
Summary
In this Flutter Tutorial, we learned how to get the value entered in a TextField.