Flutter AlertDialog – Background Color
To change the background color of AlertDialog in Flutter, set the backgroundColor property of AlertDialog with the required color.
Following is a sample AlertDialog whose background color is changed to Colors.green.
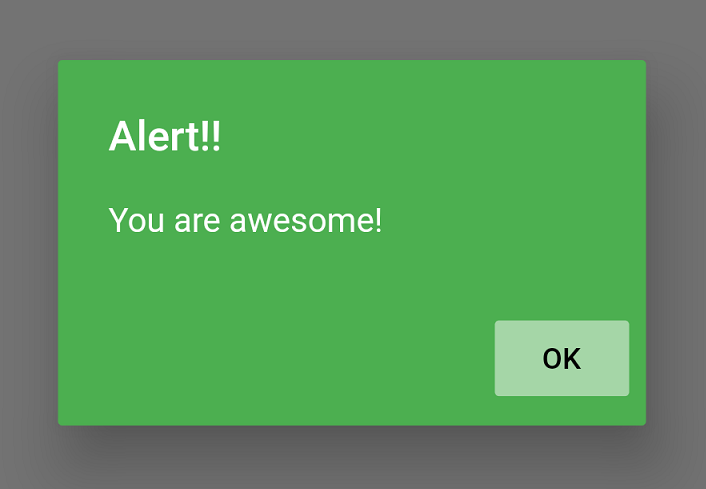
Sample code snippet to change the background color of AlertDialog widget in Flutter is
AlertDialog(
backgroundColor: Colors.green,
...
);
Example
In this example, we have created a Flutter project, and included an AlertDialog widget in our Flutter Application. We changed its background color using backgroundColor property.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Alert',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyView(),
);
}
}
class MyView extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter AlertDialog - googleflutter.com'),
),
body: Center(
child: RaisedButton(
child: Text('Alert Dialog'),
onPressed: () {
_showDialog(context);
},
),
),
);
}
}
void _showDialog(BuildContext context) {
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
backgroundColor: Colors.green,
title: new Text("Alert!!", style: TextStyle(color: Colors.white),),
content: new Text("You are awesome!", style: TextStyle(color: Colors.white),),
actions: <Widget>[
new FlatButton(
color: Colors.green[200],
child: new Text("OK", style: TextStyle(color: Colors.black),),
onPressed: () {
Navigator.of(context).pop();
},
),
],
);
},
);
}
Screenshot
Run the application on an Android Emulator and you would get the following application screen with Alert Dialog displayed in green background.

Summary
In this Flutter Tutorial, we learned how to change the background color of AlertDialog widget in Flutter application.